A deep dive into asynchronous vs synchronous programming
Learn the difference between asynchronous and synchronous programming with examples, including their pros and cons.
When we started coding and were introduced to Java and JavaScript, we all thought they were the same, right? For those who might be thinking, "What is the difference?" read till the end as I introduce you to the concepts of asynchronous and synchronous programming and the difference between asynchronous programming languages vs synchronous.
For those who already know the difference, read till the end to understand how APIs and event-based architectures are built.
What is synchronous programming?
Synchronous programming is sometimes also called linear programming.
In synchronous programming, the program is executed line by line sequentially. In a synchronous code example as shown below:
statement1
will execute fully and then move to statement2
, similarly, statement3
will only be executed after statement2
has finished executing.
It follows a blocking architecture, while one operation is being performed, other operations’ instructions are blocked. The completion of the first task triggers the next, and so on.
Characteristics of synchronous programming are:
Sequential execution - The code runs in a sequence
Statement dependency - Each statement is dependent on the previous one
I/O blocking - It can experience blocking in I/O or network operations
Synchronous programming language examples are: C and Java.
What is asynchronous programming?
Asynchronous programming, on the other hand, does not block any process and allows multiple processes to execute simultaneously.
Since in asynchronous programming, the statements do not have to wait for previous processes to finish executing, it leads to maximizing the utilization of system resources.
Here’s a diagram explaining how asynchronous programming works:

When comparing asynchronous vs synchronous programming in C#, asynchronous programming is defined by:
Concurrent – Multiple processes can run simultaneously
Event-driven – Relies on event handlers/callbacks rather than sequential code
Parallelism – Enables parallel execution on multi-core systems
Asynchronous programming language examples are: Golang and C#
Programming asynchronous applications
From the definition above, we see that asynchronous programming allows tasks to run independently without blocking the main process, which improves performance and responsiveness.
Some real-life use case examples are:
Social media feed loading: Any social media app that you use today has a feed feature where you can see recent posts from users, along with their profile pictures and other details. This is an example of asynchronous programming.
Chat applications: Any messenger or chat application that you use today and is capable of handling text messages in real time is an example of asynchronous programming.
Programming synchronous applications
In synchronous programming, processes are dependent on each other and run only after one process is complete. While this might sound like a resource-intensive process, we see synchronous programming happening in some of the tools that we use every day:
Command-line tools: As developers, we all use CLI tools, whether for running commands or installing packages from the terminal. The processes need to run one by one, only after the previous step has completed execution.
Bank ATM transactions: Let’s say you want to withdraw cash and check your account balance at the same time. This is not possible and needs to happen one after the other to avoid conflicts.
Asynchronous vs synchronous programming
From the examples above, we have seen that both asynchronous and synchronous programming are useful for different tasks.
YOU choose the method of programming depending on the task at hand.

For something like a recipe-based cooking app, where you need to follow steps one by one, synchronous programming is more useful as it follows the blocking architecture and executes one task after the other.
But for something like an online payment process, you use asynchronous programming, as it lets multiple tasks run at the same time without blocking the main process.
Both async and sync can be single-thread or multi-thread environments and are used for managing task execution, but async systems do not block threads during I/O operations.
This concept is also applicable to dynamic programming. In synchronous vs asynchronous dynamic programming, synchronous DP updates all the subproblems in a strict sequence, while asynchronous DP updates the subproblems independently.
Let us understand this with an example of an SDK. The main difference between async vs sync operations for an SDK is whether the operations will follow an execution flow or not.
Example of sync operation:
Example of async operation:
How to choose between asynchronous and synchronous programming
Choosing between synchronous and asynchronous programming depends on the kind of task you want to do.
Choose synchronous programming when you need to follow a strict sequence, while asynchronous programming is better for activities involving I/O-bound actions, such as API requests.
Other times when you can use synchronous programming are:
When the operations are CPU-bound and short.
When real-time order is preferred, like ATM and bank transactions.
When you want the code to be simple and debugging to be easy.
You can use asynchronous programming when:
Speed and responsiveness are important, like in chat applications.
You need to prioritize resource efficiency.
Multiple tasks need to run simultaneously without blocking each other, like in a social media feed.
FAQ
Is Python asynchronous or synchronous?
Python supports both asynchronous and synchronous methods of programming. For example, Flask is a synchronous web framework, while FastAPI is an asynchronous API framework.
Is Java synchronous or asynchronous?
Java is synchronous by default, but it supports asynchronous through mechanisms like threads, callbacks, etc.
Is synchronous or asynchronous better?
Which method is better depends on the kind of task you want to do. For something like a chat application, use asynchronous, while for something like terminals, use synchronous.
Is Kafka synchronous or asynchronous?
Kafka is an asynchronous tool.
Is JavaScript asynchronous or synchronous?
JavaScript is synchronous, but it can handle asynchronous tasks through methods like `async/await`, which is important when discussing asynchronous vs synchronous programming in JavaScript.
This article was first published on February 3rd, 2023, and was improved by Haimantika Mitra as of January 17th, 2025, to improve your experience and share the latest information.
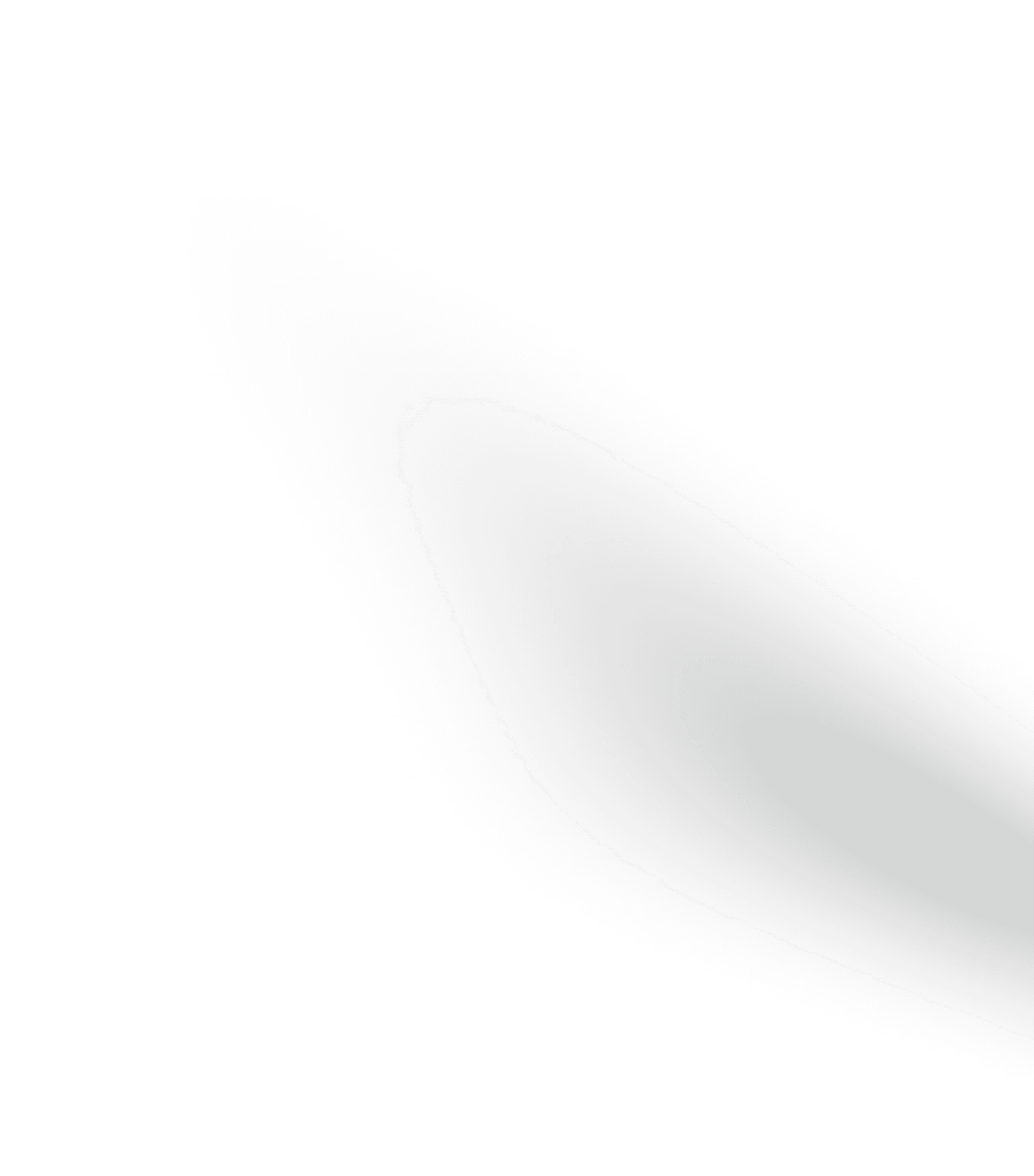