The Guide to VS Code Snippets
Learn about VS code snippets, and how to create them in your editor. Plus, how Pieces makes saving, using, and sharing VS code snippets even easier.
A software developer’s job mainly involves writing production code. It’s easy to find yourself typing the same lines of code repeatedly as you work. This repetitive and inefficient task can certainly begin to feel mundane after a while, and as a result, your coding workflow may start to look like a chore. One solution to this problem is to use code snippets to speed up and simplify the coding process. In this article, you’ll learn more about code snippets and how to create them in the Visual Studio (VS) code editor. In the end, you’ll also see how Pieces for Develoeprs makes saving, using, and sharing VS code snippets even easier.
What are Code Snippets?
Code snippets are ready-made bits of reusable source code that you can save for reuse as needed. With code snippets, you can quickly insert the chunks of reusable code into your codebase, reducing the time you would spend repeatedly typing the same code and thus significantly speeding up your development workflow.
Generally speaking, it’s preferable to avoid repetition when there’s an obvious solution: You save contacts on your phone instead of typing the numbers for every phone call, and you let websites store cookies on your computer to remember your log-in details and preferences. Using Visual Studio Code snippets follows this same line of thought.
Here are a few specific reasons code snippets can be helpful to your development workflow:
Increase Development Speed
Imagine building a website of ten landing pages using HTML5. Typing the HTML document boilerplate for each page would be daunting and, frankly, a waste of your time.
With code snippets in Visual Studio, you write the code and save it as a snippet. Then, you can easily reuse the snippet on each page to write the boilerplate in a templated fashion.
This way, you save yourself from unnecessary amounts of keystrokes, giving you the time to focus on other development needs. Especially when it comes to large blocks of code, it’s easy to see how using code snippets can be a significant productivity booster.
Prevent Typing Errors
Using code snippets also leaves little room for typographical errors. When repeatedly typing the same thing manually, you’re bound to make a mistake at least once. However, code snippets help you avoid such errors by providing a method to ensure consistent and fault-free syntax across your codebase since you have to type the code only once and can then reuse it multiple times.
Make Coding More Enjoyable
Finally, typing the same lines of code can make your software development workflow feel dull and mundane. Using code snippets helps to eliminate repetitive tasks, freeing up your mental resources to focus on writing enjoyable code that actually interests you.
How to Create Code Snippets in VS Code
Now that you’re aware of the benefits code snippets provide to developers, let’s take a look at how you can create and use VS Code user snippets.
Most code editors and IDEs have out-of-the-box support for code snippets, and VS Code is no exception. Follow the steps in the tutorial below to get started.
Step 1: Create a Snippet File
VS Code snippets are written in JavaScript Object Notation (JSON) files that can define an unlimited number of snippets. To create a snippet, start by opening the command palette. Depending on your operating system (OS), you can do that using the command Ctrl+Shift+P on Windows or CMD+Shift+P on a Mac. Type the word “snippet” into the search bar and toggle the Preferences: Configure User Snippets option.
This will present you with a drop-down of different languages and selections to choose from:
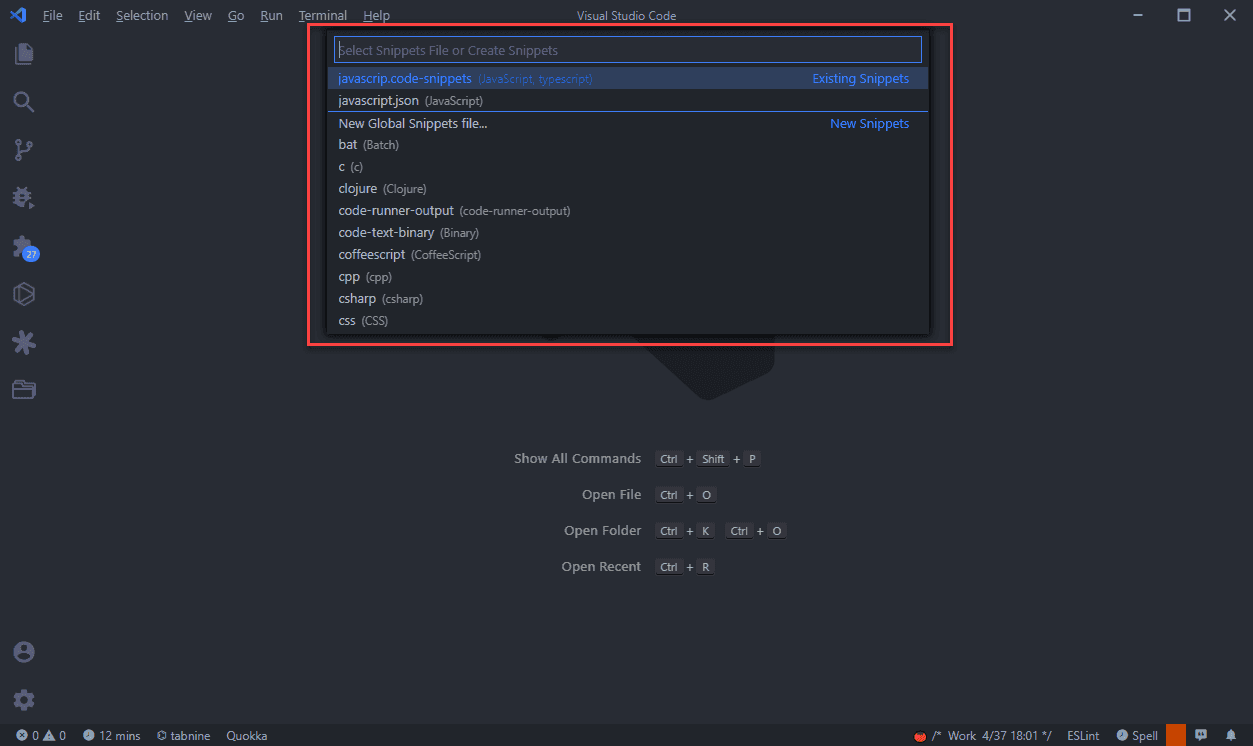
Step 2: Define Your Snippet’s Scope
Next, you’ll need to define the snippet’s scope, which refers to the languages the snippet will be available in. VS Code supports two types of scopes:
Global scope snippets: You can use these code snippets across several or all languages of your choice.
Language-specific scope snippets: You can use these code snippets only in a particular language.
For this tutorial, you will be creating VS Code Global snippets. From the drop-down list, select the New Global Snippets file option and then give the snippet file a name:

With that done, VS Code will create a new snippet file that includes a few comments and an example snippet, as pictured here:
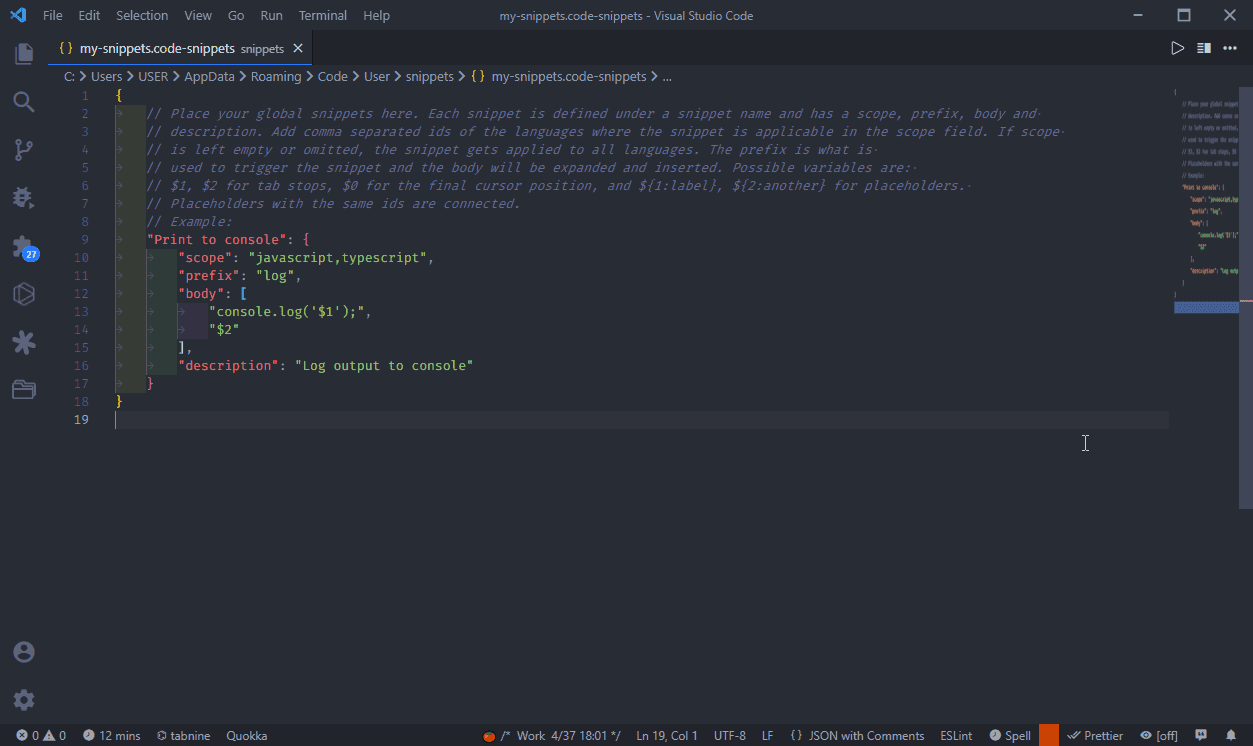
Step 3: Write the Snippet’s Syntax
The example snippet of code above is a sample template to show you the format for how to create your own snippet. Line by line, here’s an overview of what each field does:
Print to console: This is the snippet’s name, which will also be displayed via IntelliSense if no description is provided. It is the property of the JSON object that will contain the actual details of the snippet.
Scope: This determines the languages allowed to use the snippet. You can specify as many languages as you wish, separated by commas. If the scope is omitted, the snippet can be accessed from any language. In this example, the snippet is scoped to only JavaScript and TypeScript.
Prefix: This defines one or more words you need to type for the snippet to appear. In the example, you need to type “log” to display the snippet.
Body: This is where you define the code snippet. It can be a string if it is a single line of code or an array of strings if it has multiple lines, where each string forms a new line in the snippet. In this example, the actual code is
console.log
.$1
and$2
: These are called tab stops. They enable you to pinpoint where the cursor should be placed within the code snippet after being injected and also allow you to tab through the code easily using the tab key.$1
is simply a placeholder for where the cursor should be initially placed after invoking the snippet, and$2
is the next point the cursor placement should move to after hitting the tab key.Description: This describes VS code snippets by allowing you to provide details on what it’s all about. If it is omitted, the name of the snippet will be used instead. In this example, the description tells you that the snippet’s purpose is to “log output to console.”
Now that you have an example code snippet saved, let’s take a look at how you can use it in your workflow.
Using VS Code Snippets in Your Workflows
There are several ways you can use this snippet in your actual development workflow. Here’s a quick overview of a few of them.
Using VS Code IntelliSense
VS Code IntelliSense is the easiest way to use code snippets. It automatically aids you by providing autosuggestion and autocompletion, among several other features. To use VS Code IntelliSense, start by typing the prefix for the snippet. This will display a context menu with hints at what you might be trying to achieve:
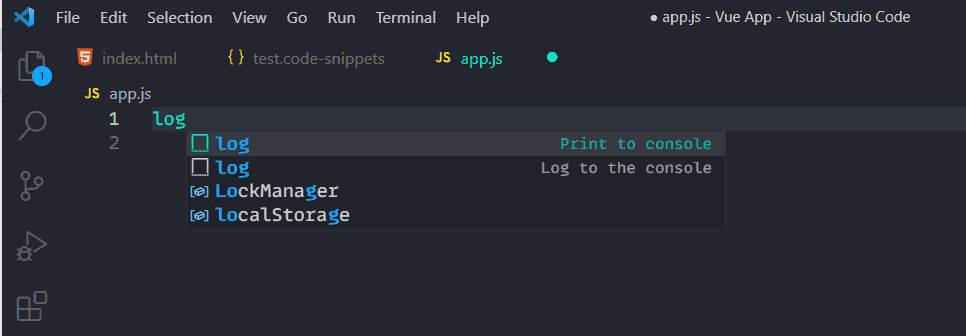
You can then select the code snippet by hitting the tab key to automatically insert it into your code:
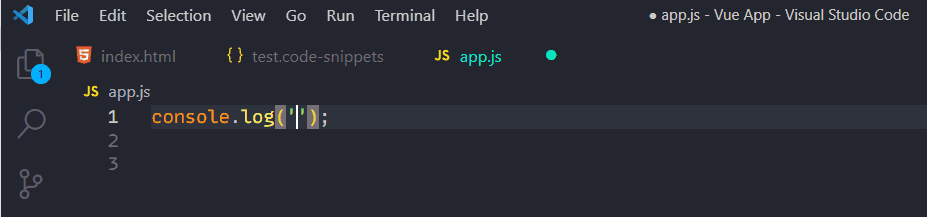
As you can see, the cursor is immediately placed where the `$1` placeholder was in the snippet’s file.
Using the Snippet Picker
Another method to use snippets in your code is with VS Code’s Code Snippet Picker. Open the command palette and type “insert” into the search bar. Then select the Insert Snippet option.
This displays a drop-down list of all the custom VS Code snippets you have created:
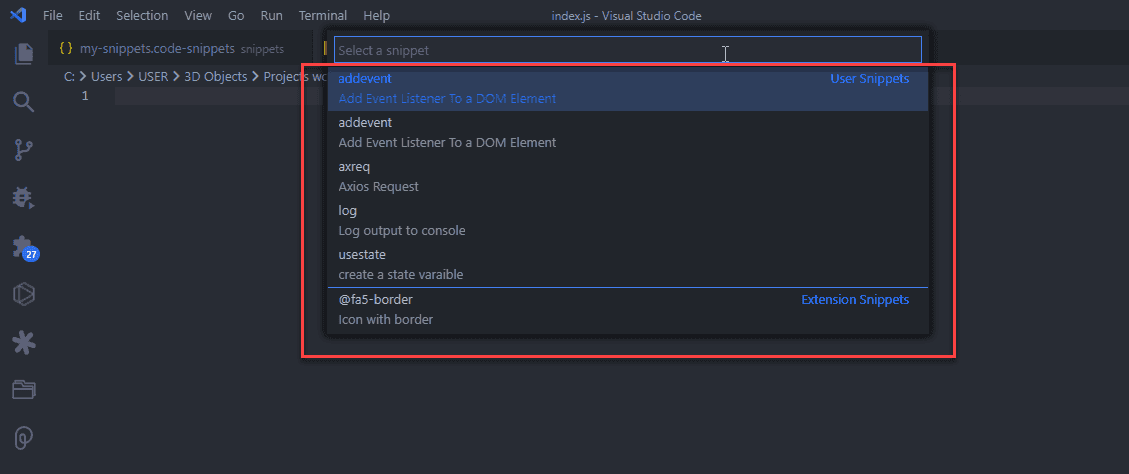
From here, you can select the appropriate snippet, and it will be inserted into your code.
Using Pieces — a Better Way to Save Code Snippets
As your snippets grow in lines and complexity, it can become difficult to go through the process of creating them in VS Code. Enter Pieces for Developers, the perfect code snippets plugin to solve that problem.
Pieces is an artificial intelligence (AI) tool that allows you to instantly save code snippets from your web browser, code editor, and even images. Pieces for Developers makes the process of creating, finding, and using snippets a breeze.
Creating VS Code Snippets with Pieces
To start creating code snippets with Pieces, download Pieces for Developers for your OS and install the integrations for your code editor and web browser.
On a web browser, hover your cursor over any code block to display the Pieces buttons. Clicking on that widget allows you to save that block of code to Pieces:
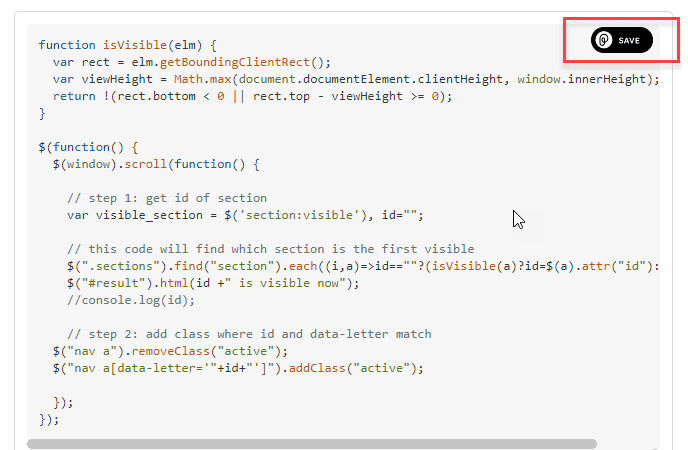
In your code editor, highlight the snippet with your mouse, right-click to open VS Code’s context menu, and click on Save to Pieces to save the snippet to Pieces:
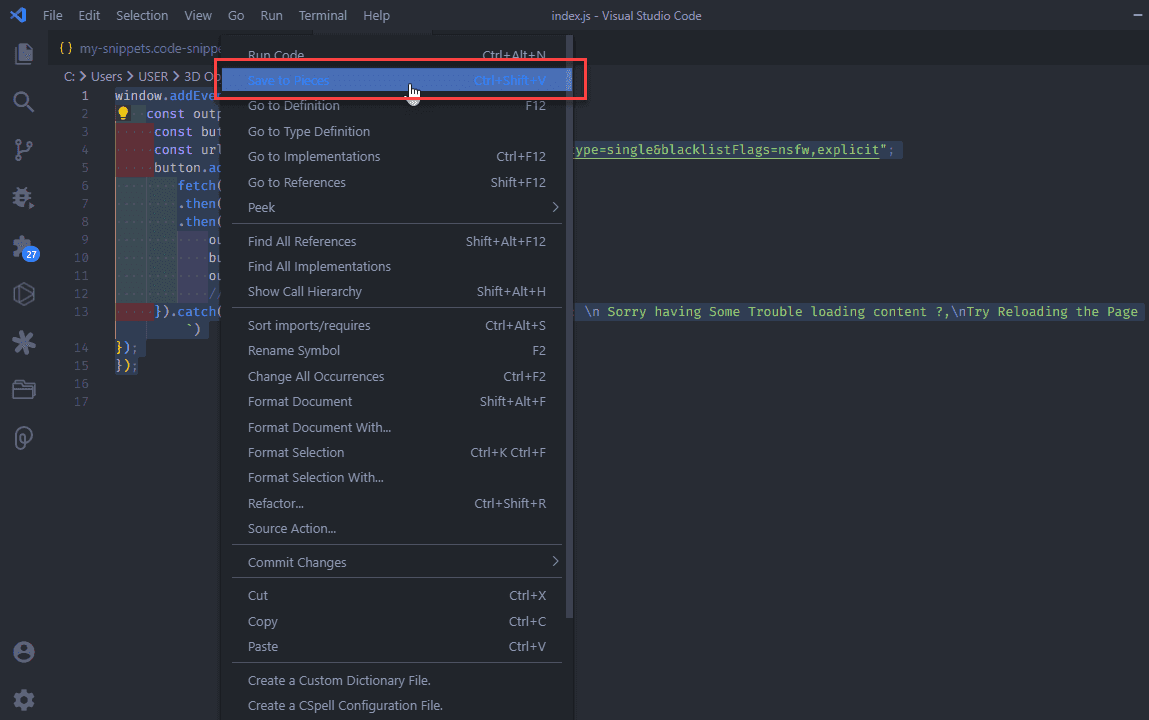
With your snippets saved in Pieces for Developers, you can easily reuse them by clicking on the cascading-windows icons on the Pieces app sidebar to copy the snippet to your clipboard and then paste it into your codebase.
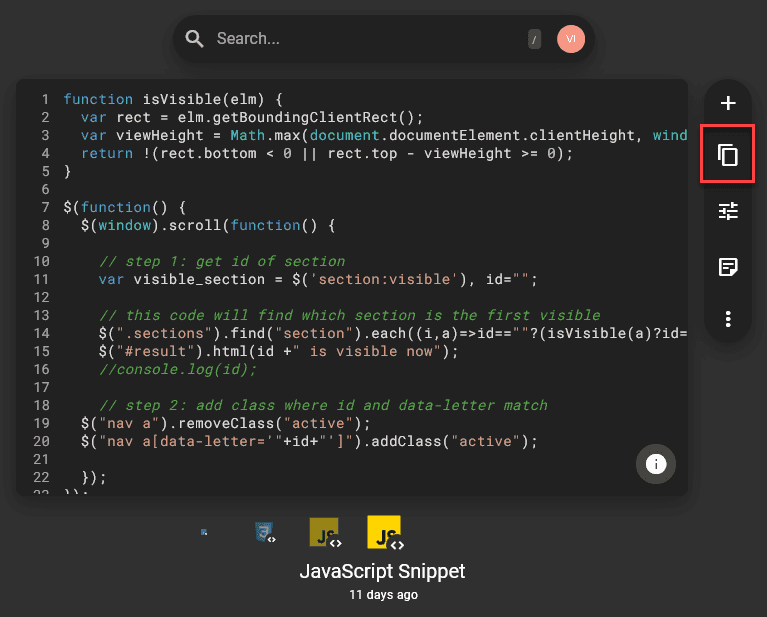
Pieces offers a ton of unique features, such as auto-formatting your snippets; categorizing them by language and by tags to enable you to quickly search for them; capturing the project, file name, and line numbers that the snippet came from in your editor; storing the URL that the snippet came from if you saved it from a web page; and many more.
Conclusion
Developers tend to carry out a lot of repetitive tasks during the course of development, which can really undermine efficiency when writing code. Using code snippets lets you focus on the right things while writing code and eases your entire development workflow.
In this article, we learned how to create VS code snippets, as well as how to optimize your workflow using Pieces for Developers. To learn more, get started with Pieces for free today!
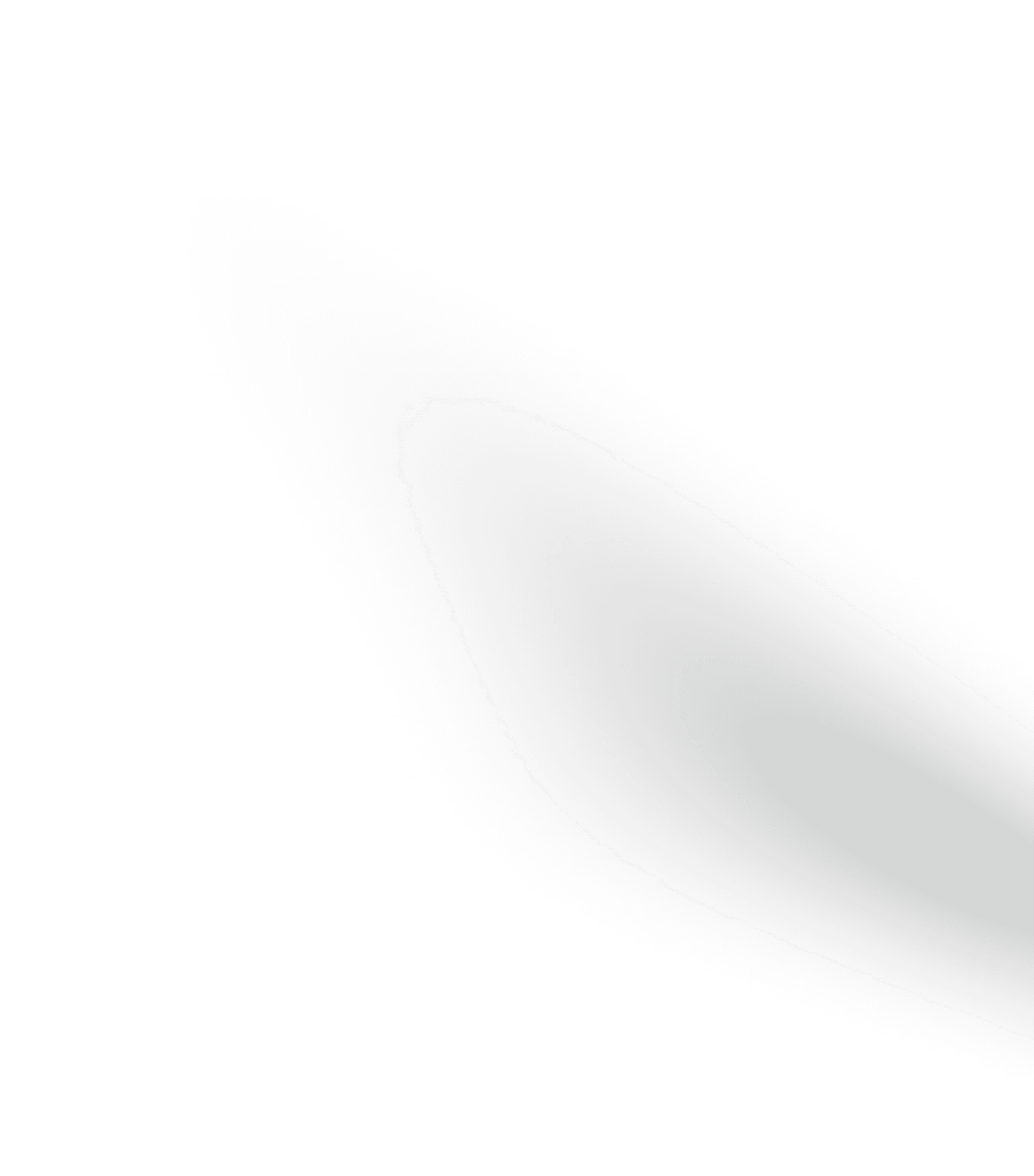